Overview
This Project Portfolio documents my contributions to MooLah, a project made in NUS' Software Engineering module.
My team, consisting of 5 Computer Science students, was tasked to enhance an existing project, Address Book Level 3. Over the course of the semester, we morphed the original Address Book code into MooLah, an expense tracker that accepts input through the Command Line Interface (CLI). We found many existing expense trackers on the market to be relatively troublesome and hence sought to create a hassle-free expense tracker specifically tailored to NUS students. MooLah has a clean and efficient Graphic User Interface (GUI) made from JavaFX, and supports many quality-of-life features such as autocompletion of input. At its core, MooLah allows users to easily manage their spending with the use of budgets, expenses, events and robust statistics calculations.
Summary of contributions
The following is a summary of my contributions to MooLah. I was mainly responsible for the development of events and time-based features.
-
Major enhancement: I added the ability to track events in MooLah.
-
What it does: This feature allows the user to add, edit and delete events, which are the user’s important future activities that they have to spend money on (e.g. a friend’s birthday). MooLah reminds the user of upcoming events, and on the day of the event, will automate the adding of the corresponding expense from the event.
-
Justification: Many of our users lead busy lives and may sometimes forget to input expenses from important events that they attend, or may forget these events altogether. My feature solves this issue by letting users input a future event; MooLah then automates the reminding and the eventual adding of the expense from the event. Such a 'fire-and-forget' implementation greatly enhances convenience for the user.
-
Highlights: Automating the reminding and adding of expenses from events requires MooLah to keep constant track of system time. I also had to work closely with my teammate who implemented the budgeting feature as every event, and its corresponding expense, had to fall under the correct budget.
-
-
Minor enhancements: I implemented several time-based features in MooLah.
-
Fully implemented the Timestamp field into MooLah’s expenses and events, hence allowing users to keep track of when exactly their expenses and events happen.
-
Implemented constant system-time checking in MooLah via the use of a timer that runs on a parallel thread. This allows MooLah to accurately refresh budgets and enables precise timekeeping of expenses, events and budgets. (#132)
-
Integrated, with some modifications, the third-party Natty library into MooLah. This allows MooLah to support natural language date-time parsing. (#132)
-
Improved the parsing and validity-checking of the Price field. (#216)
-
-
Other contributions:
-
Project management:
-
Oversaw code quality across the project. (i.e. made sure code adhered to good software engineering principles) (Example).
-
Managed and assigned issues that arose from the bug-testing session of the Mock Practical Examination.
-
-
Testing:
-
Refactored existing tests to include the newly added Timestamp field of expenses.
-
-
Documentation:
-
Wrote the DateTime parsing and Events section of the User Guide.
-
Improved the Expenses section of the User Guide.
-
Wrote the Non-functional Requirements, Glossary, and Product Survey sections of the Developer Guide.
-
Added use cases for events in the Use Cases section of the Developer Guide.
-
-
Community:
-
Contributions to the User Guide
Given below are my major contributions to MooLah’s User Guide. They showcase my ability to write documentation targeting end-users. |
Natural language Datetime parsing
In MooLah, you can type all dates and times in natural language! You no longer have to remember what date it was a week ago; just type '1 week ago' into the appropriate parameter in the command line and MooLah will handle the rest! Datetime formats are parsed mostly by a third-party Natural Language Parser library, Natty, which supports human jargon. Please refer to http://natty.joestelmach.com/ for documentation and more information.
Natty naturally parses all datetime formats in the American format (e.g. MM/dd, MM-dd). In MooLah however, we have modified the parser such that all formats are parsed in the international format (e.g. dd/MM). So, the tryout page on Natty’s official website will parse dates differently in this aspect. |
Event-related Commands
Events are pretty similar to expenses, except that they denote potential expenses that may happen in the future. You should use this feature to keep track of future important events in your life that would require you to spend some money (e.g. your friends' birthdays).
At launch, MooLah will remind you of your upcoming events. While using MooLah, any transpired events also appear as popups, prompting you whether you wish to add these events as corresponding expenses.
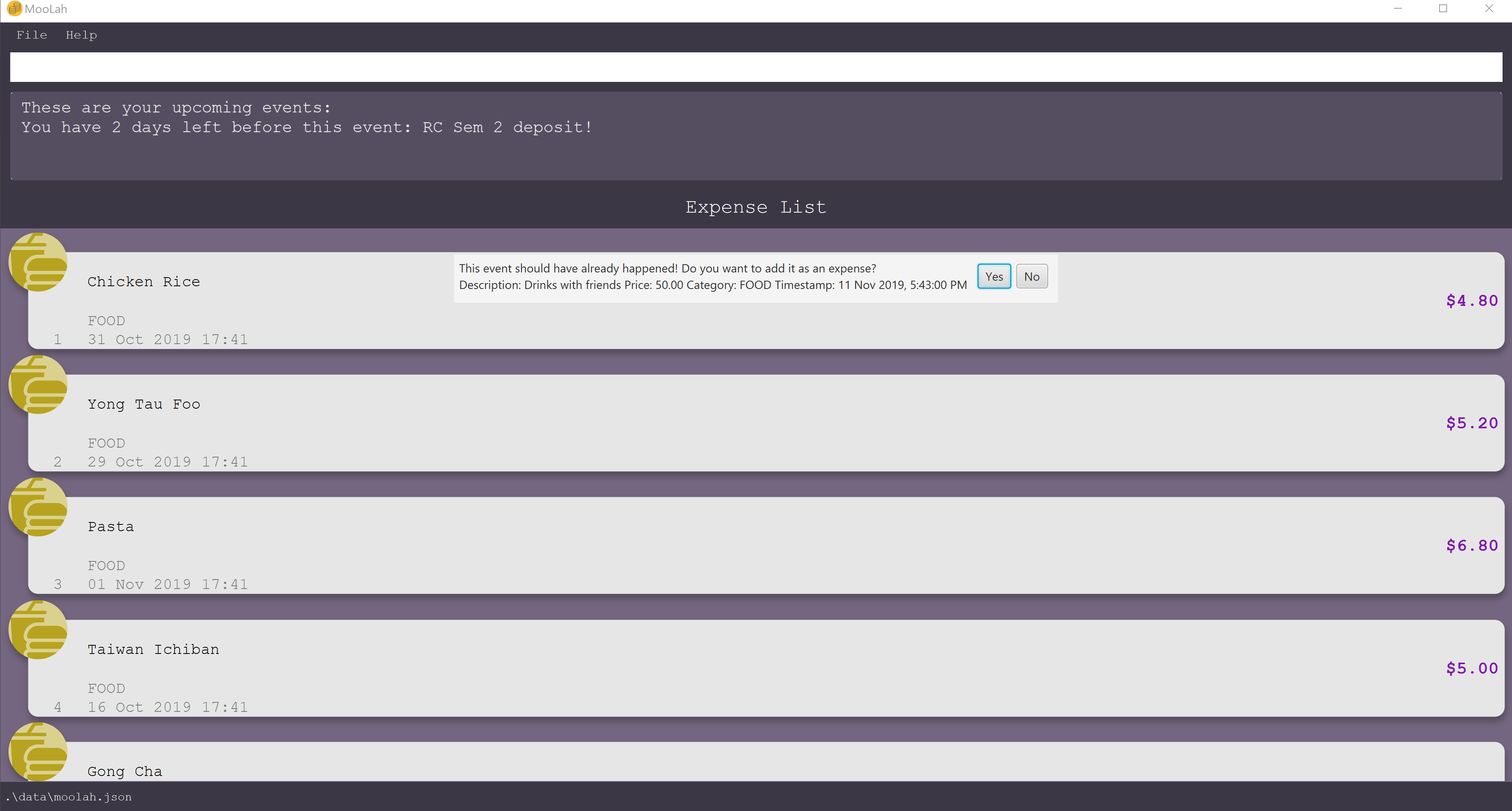
Once an event has transpired, it is permanently deleted from MooLah and you cannot get it back! Undoing MooLah to a state where that event was still present would not restore the event. |
MooLah checks for transpired events every 2 seconds, so you may experience a very slight delay when it comes to
event popups! A popup would also not appear if the transpired event has had its corresponding budget deleted. |
Adding an event: addevent
Events share the same fields as expenses, so you add them in the same way, albeit with a different command word.
Format:
addevent d/<DESCRIPTION> p/<PRICE> c/<CATEGORY> t/<TIMESTAMP>
Examples:
-
addevent d/Brian birthday p/40 c/Shopping t/two weeks from now
-
addevent d/Family buffet c/Food p/250 t/31-12
-
addevent d/Bangkok plane tickets t/tomorrow p/200 c/Travel
Listing all events : listevent
You can list every single event currently tracked by MooLah.
Format:
listevent
Updating an event: editevent
You can edit events the same way you edit expenses.
Format:
editevent <INDEX> [p/<PRICE>] [d/<DESCRIPTION>] [t/<TIMESTAMP>] [c/<CATEGORY>]
Example:
listevent editevent 2 p/300
This will update the price of the 2nd event in the current list to be 300.
Deleting an event: deleteevent
You can also delete an event from the events list in MooLah.
Format:
deleteevent <INDEX>
Examples:
listevent delete 2
This will delete the 2nd event in the list of events, shown by listevent
.
Contributions to the Developer Guide
Given below are my major contributions to MooLah’s Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Events feature
Implementation
The Events feature allows users to add events that are supposed to occur on a future date.
On launch, MooLah will remind users of upcoming events. While the app is open, MooLah will also notify the user about any events that have transpired, and allow them to automatically add these events as expenses.
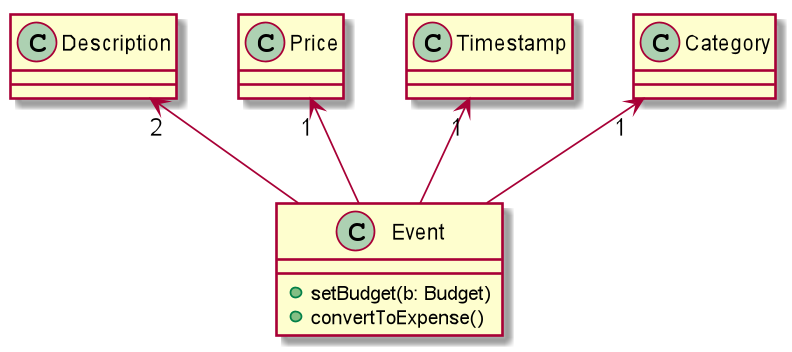
Like expenses, events hold two Descriptions (one for its details and one for the budget it belongs to), a Price, a Timestamp
and a Category. The setBudget
method of Event is used for the Event to remember which budget it was added to. This facilitates
the potential conversion of the Event into an Expense through the convertToExpense
method. This method is called when the user
accepts the automated addition of an expense from the corresponding event, into the budget that the event was added into a while back.
Since Event’s fields are a subset of that of Expense, all its fields are passed into the Expense constructor during the conversion, and a
unique identifier is then generated to complete the creation of the expense, allowing it to be added to MooLah.
The Events feature supports the addevent
, deleteevent
, editevent
and listevent
command words.
As mentioned earlier, MooLah displays reminders of upcoming events during launch. The implementation is shown below:
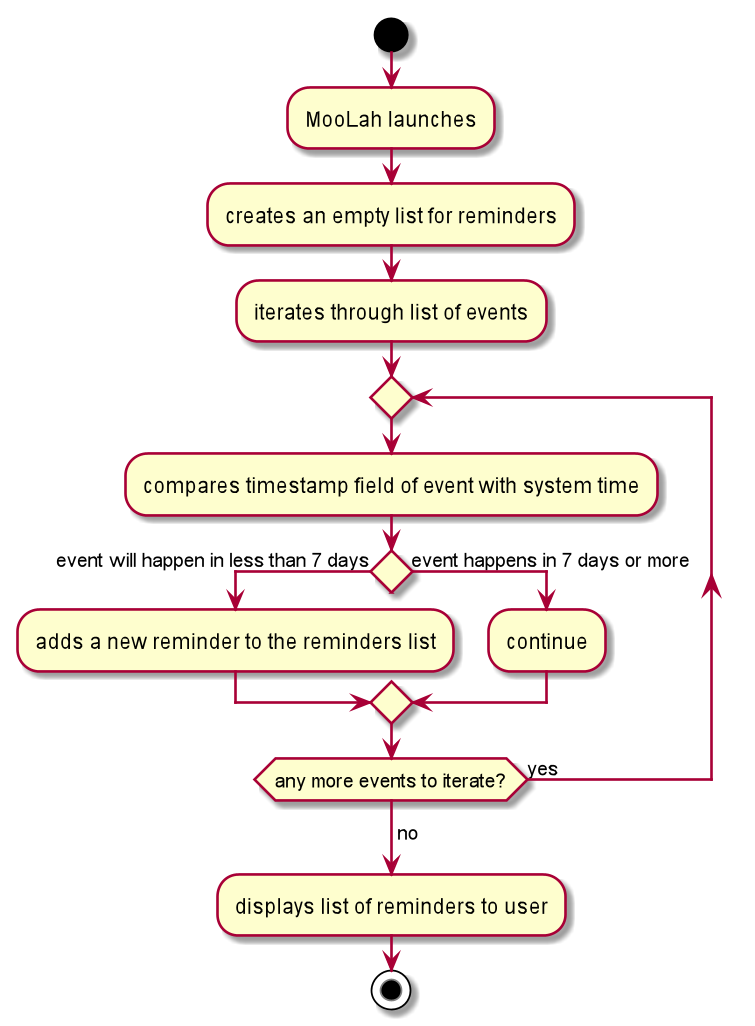
To allow for precise timekeeping of expenses and events as well as accurate prompting of transpired events, MooLah tracks system time every 0.05 seconds using a Timer
running
on a parallel thread. Using another Timer
, MooLah then checks for transpired events every 2 seconds; an event is then deemed to have transpired if its timestamp has gone past system time.
The following sequence diagram shows how MooLah handles transpired events:
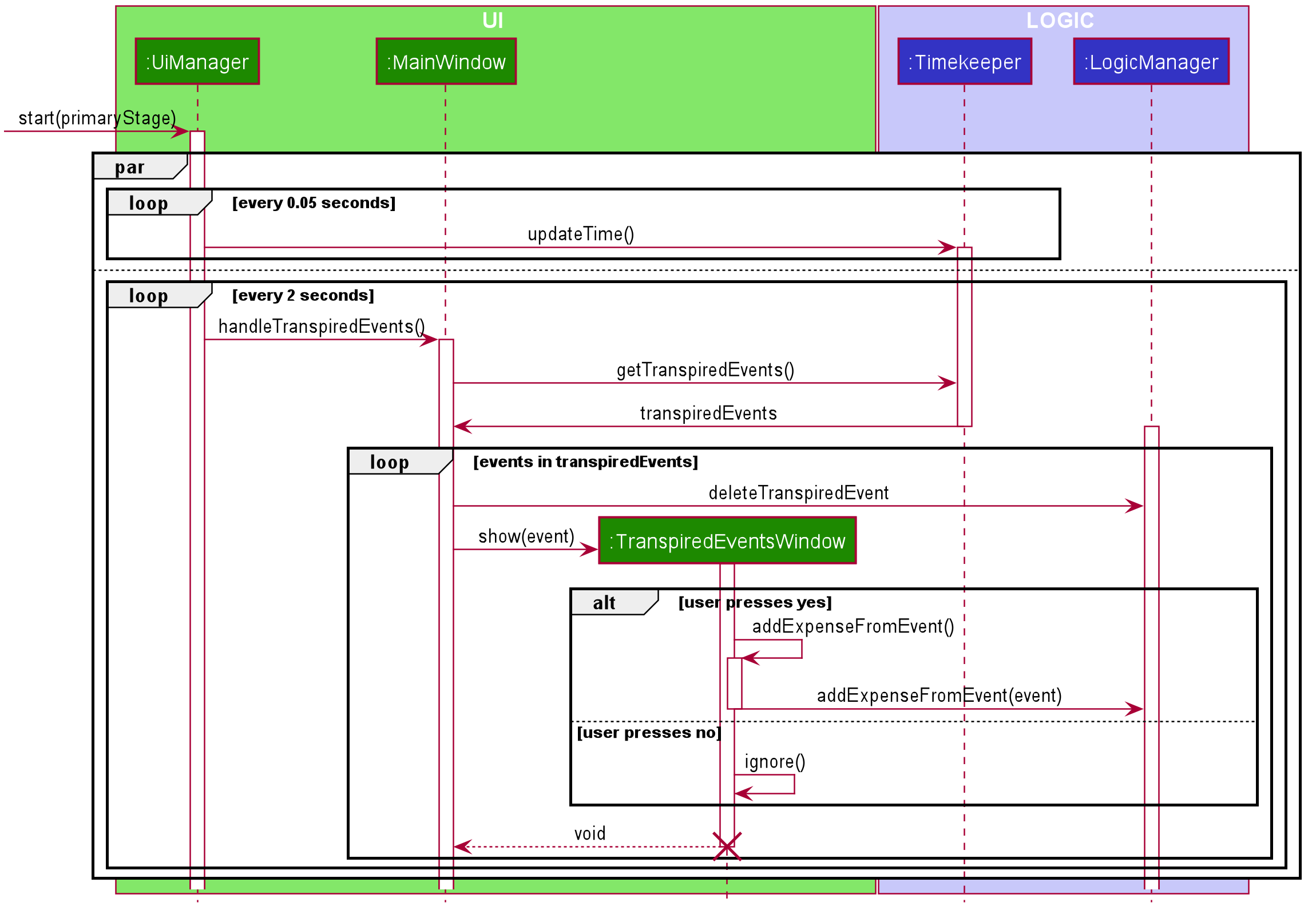
-
When MooLah launches, the
start
method ofUiManager
is called. Every 0.05 seconds,UiManager
callsupdateTime
of Timekeeper to update system time. Concurrently,UiManager
also calls thehandleTranspiredEvents
ofMainWindow
every 2 seconds, which fetches all transpired events and notifies the user about them. It does so by first obtaining the transpired events from theTimekeeper
class. -
Once the transpired events have been obtained, for each transpired event, a new
TranspiredEventWindow
will be constructed. The transpired event is deleted from the model and will then be passed viashow
, a method of the newly constructedTranspiredEventWindow
.show
will create a popup window that details the transpired event, and asks the user whether it should be added as an expense. This popup window will have a 'Yes' and 'No' button. -
If the user presses the 'Yes' button, the event is passed to the
addExpenseFromEvent
method ofLogicManager
, where it is then converted into its corresponding expense, and a command to add the expense is run. -
If the user presses the 'No' button, the expense is not added.
-
After pressing either button, the popup window closes.
Design Considerations
Aspect: What command words should the Events feature use?
-
Alternative 1:
addevent
,deleteevent
, etc.-
Pros: Makes it clear to the user that events are separate from expenses
-
Cons: Somewhat clunky and redundant, especially since expenses and events share the same fields
-
-
Alternative 2:
add
,delete
, etc. (i.e. same command words as adding expenses)-
Pros: More streamlined, makes use of the fact that expenses and events share the same fields
-
Cons: The distinction between events and expenses is more blurry to the user
-
-
Solution (Current Implementation): Adopt Alternative 1. Although Alternative 2 is more intuitive, it is not suited to our current implementation of generic command words. Since command words such as
add
anddelete
now result in different commands based on which panel the user is on, MooLah’s parser would not know whether users want to add an event or expense if they typeadd
while on an event or expense panel. This necessitates the splitting of expense and event commands into two separate command words.
Future Implementation (Coming in v2.0)
Users can set their expenses to recur. Recurring expenses will generate events daily/weekly/monthly (based on the user’s decision) that can then be added as expenses when their due dates are reached.